The Hypertext Transfer Protocol (HTTP) is the foundation of data communication on the web, enabling the transfer of information between clients and servers. Each time a web browser or API makes a request to a server, the server responds with an HTTP status code that indicates the outcome of that request.
Understanding HTTP status codes is essential for anyone involved in web development or API design. These codes help diagnose issues, optimize user experience, and ensure smooth interactions between services. Whether you're building websites, APIs, or managing microservices, knowing what these codes mean can save you significant time when troubleshooting and help you design better, more resilient applications.
In this blog, we’ll focus on error codes, particularly 4xx (client-side errors) and 5xx (server-side errors), as they are critical for identifying and resolving problems in web and API development. While success codes like 2xx and redirection codes like 3xx have their importance, error codes often demand more attention due to their immediate need for resolution and impact on user experience.
Let's dive into some of the most common error codes and what they mean for developers and users alike.
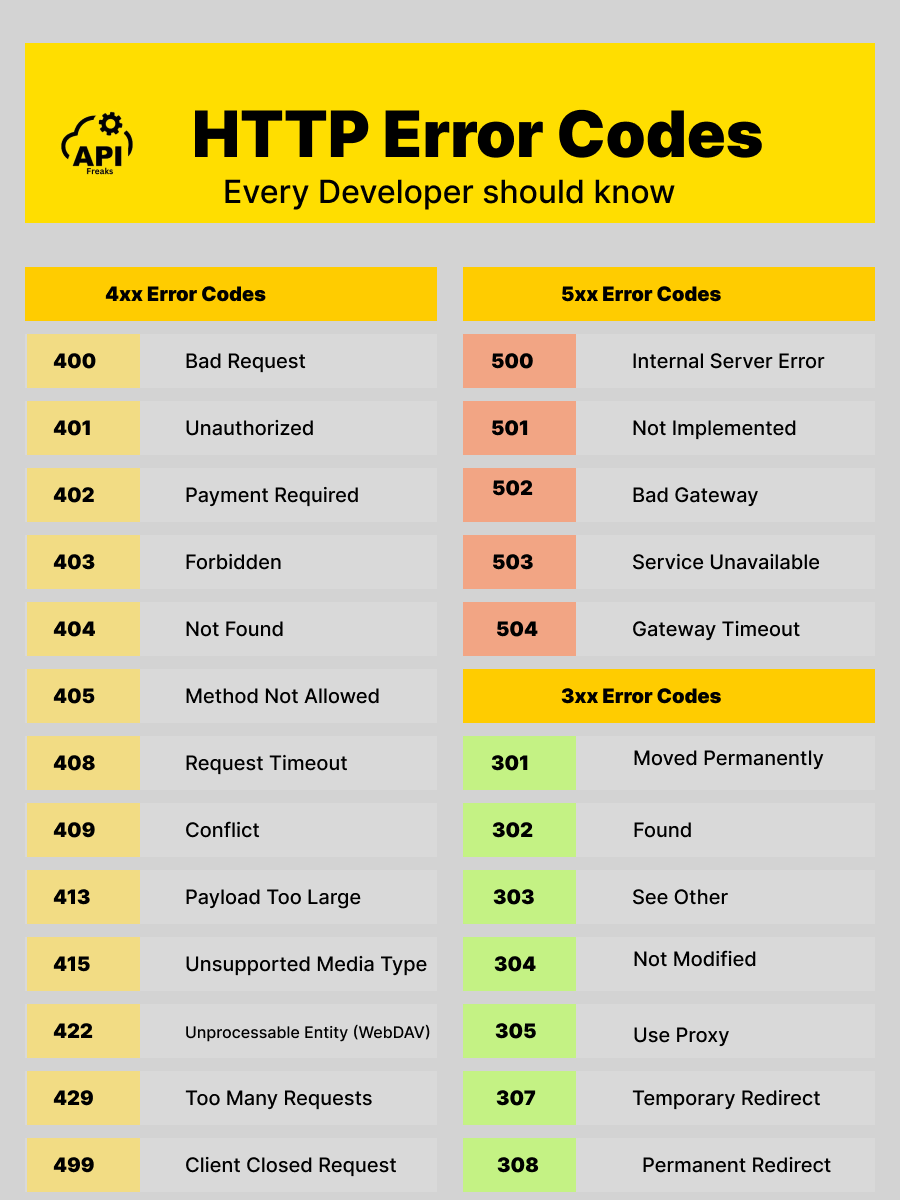
4xx Error Codes
400 - Bad Request
The 400 Bad Request error occurs when the server cannot understand the request due to malformed syntax. This usually means there’s an issue with the client-side input, such as incorrect formatting, missing required parameters, or improper encoding.
Common Causes:
- Invalid URL or request structure: Sending requests with incorrect or poorly structured syntax.
- Incorrect JSON formatting: If an API expects JSON but receives improperly formatted JSON, a 400 status code is returned.
- Missing required fields: In API requests, failing to include necessary fields in the payload can trigger a 400 error.
- Unsupported or invalid characters: Special characters in URLs, headers, or payloads that the server cannot process.
- Outdated browser cache: An outdated browser cache can lead to malformed requests and 400 errors.
Handling Strategies:
- Validate input on the client-side: Before making a request, ensure that the input data is properly validated and meets the server’s expectations (e.g., correct formats, no missing parameters).
- Provide detailed error messages: When developing APIs, make sure the server returns informative error messages that help the client identify and fix the issue.
- Use tools for debugging: Utilize API testing tools like Postman or cURL to debug and inspect the request structure. This helps developers pinpoint malformed syntax and quickly resolve issues.
- Check for API documentation: Always ensure that the client adheres to the API’s specification, which can help prevent common 400 errors related to parameter structure and data types.
- Clear the browser's cache: Clearing the browser's cache can resolve potential issues caused by an expired or corrupted cache.
401 - Unauthorized
The 401 Unauthorized error indicates that the client request lacks valid authentication credentials for the target resource. Essentially, the server is unable to verify the user’s identity, either because no credentials were provided or because they were incorrect. To resolve this, clients must provide valid authentication credentials.
Common Causes:
- Missing or invalid authentication token: When making requests to secured APIs, failing to include a valid token or providing an expired one often results in a 401 status.
- Incorrect login credentials: If the client sends wrong username/password pairs, the server will reject the authentication.
- Token expired or revoked: OAuth tokens or session cookies may expire or be revoked, leading to unauthorized access.
- Missing access token: Ensure that a valid access token is included in the request headers to gain access to the resource.
Handling Strategies:
- Ensure valid credentials: Always check that your authentication token is active and correctly formatted. Refresh or re-authenticate if tokens are expired.
- Use proper authentication methods: Ensure that the correct authentication method (e.g., Bearer tokens, Basic Auth) is applied when making API requests.
- Check API permissions: Ensure that the user has the necessary permissions to access the resource. Consult the API documentation to verify authentication methods and required scopes.
- WWW-Authenticate header field: The server must include the WWW-Authenticate header field in its response to guide clients on how to provide valid credentials for accessing the target resource.
402 - Payment Required
The 402 Payment Required status code is reserved for future use, mainly in cases where payment is required to access a resource. While not commonly encountered in many APIs today, it’s intended to be used in scenarios where resources are behind a paywall or subscription model.
Common Causes:
- Subscription required: Accessing premium features or data may require a payment or subscription, and failure to meet these requirements will result in a 402 error.
- Payment processing issues: If the payment for a service fails or is incomplete, the server may return a 402 response to prompt the user to complete the transaction.
- Rate limits tied to payment tiers: Some APIs have usage limits based on paid plans, and exceeding free-tier limits without upgrading may trigger this error.
Handling Strategies:
- Check payment status: Ensure that the client has an active subscription or that the payment has been successfully processed.
- Implement clear messaging: When building an API, provide a clear error message explaining that payment is required or that the user has reached a limit.
- Allow easy payment upgrades: If applicable, provide users with a straightforward way to upgrade their plan or process payment to resolve the issue.
403 - Forbidden
The 403 Forbidden error occurs when the server understands the request but refuses to fulfill it. This is usually due to the client not having the necessary permissions to access the resource.
Common Causes:
- Insufficient permissions: The client’s authentication credentials may not grant access to the requested resource.
- Access control settings: The server might restrict access to certain users or roles based on their permissions or access level.
- IP restrictions: In some cases, the server may block requests based on IP address, resulting in a 403 error for unauthorized clients.
- Resource restrictions: The resource may be available only to certain users, and attempts to access it without the required authorization triggers this error.
Handling Strategies:
- Verify user permissions: Ensure the client has the correct access rights for the resource. For APIs, check if the authentication token has the required scopes.
- Provide helpful error messages: When developing an API, return detailed 403 error messages indicating why access is denied, such as missing permissions or restricted roles.
- Check IP restrictions: If applicable, verify if the client’s IP address is allowed by the server or API.
404 - Not Found
The 404 Not Found error occurs when the server cannot find the requested resource. This is one of the most common HTTP errors and typically means that the URL is incorrect or the resource has been moved or deleted.
Common Causes:
- Incorrect URL: The client may have mistyped the URL, or the path may be incorrect.
- Resource moved or deleted: The requested resource no longer exists or has been relocated without a proper redirect.
- Broken links: Outdated links that point to nonexistent pages or resources.
- Missing routes in APIs: In API development, requesting an endpoint that hasn’t been defined will result in a 404 error.
Handling Strategies:
- Check and correct the URL: Ensure the URL is typed correctly and points to an existing resource.
- Implement proper redirects: If a resource has been moved, implement 301 redirects to point users to the new location.
- Keep API documentation updated: Ensure that API clients use the correct endpoints as per the API documentation, and remove or update deprecated routes.
405 - Method Not Allowed
The 405 Method Not Allowed error occurs when the request method (e.g., GET, POST, PUT, DELETE) is not supported by the server for the requested resource. The client might be trying to use a method that is not allowed or implemented for the specified endpoint.
Common Causes:
- Incorrect HTTP method: Trying to use POST when only GET is allowed, or vice versa.
- Unsupported API operation: The API may not support certain methods for specific resources.
- Misconfigured routes: Server routes may not be configured to accept all HTTP methods, leading to a 405 error.
Handling Strategies:
- Check API documentation: Ensure you are using the correct method as outlined in the API specification.
- Implement proper error handling: If developing APIs, clearly define which methods are allowed for each endpoint and return helpful messages to guide clients.
- Route configuration: Ensure that server-side routes are configured to support all necessary HTTP methods for the requested resources.
408 - Request Timeout
The 408 Request Timeout error indicates that the server did not receive a complete request from the client within the time it was prepared to wait. This error usually occurs when the client takes too long to send a request, and the server times out the connection.
Common Causes:
- Slow client connection: Poor internet connection or server-side timeouts.
- Large payloads: Sending large files or data may cause delays that exceed the server’s timeout limit.
- Network issues: Network instability between the client and server can lead to delayed requests.
- Server overload: If the server is under heavy load, it may take longer to process incoming requests, resulting in timeouts.
Handling Strategies:
- Optimize client requests: Ensure that requests are sent promptly without unnecessary delays. This can involve optimizing large payloads or sending them in chunks.
- Increase server timeout settings: If the server frequently experiences timeouts due to slow connections, consider increasing the server’s timeout threshold.
- Monitor network conditions: Use tools to detect and resolve network-related issues that might be causing timeouts.
- Implement retries: For API requests, implement retry mechanisms to automatically resend a request if it times out.
409 - Conflict
The 409 Conflict error occurs when the request conflicts with the current state of the server. This error is typically encountered when multiple requests are trying to modify the same resource in a way that results in a conflict. In APIs, this could be due to editing the same data or attempting actions that contradict the server’s existing state.
Common Causes:
- Version control conflicts: When multiple users or systems try to update the same resource simultaneously, resulting in conflicting changes.
- Duplicate resource creation: Trying to create a resource (e.g., a record in a database) that already exists, based on unique constraints.
- State conflicts: Attempting to perform operations that are inconsistent with the current state of the resource (e.g., trying to delete something that doesn’t exist).
Handling Strategies:
- Implement versioning: Use resource versioning to avoid conflicts in environments where multiple systems or users update the same resource.
- Return informative error messages: The server should provide clear error messages indicating what caused the conflict and how to resolve it.
- Handle retries: When appropriate, clients can handle this error by retrying the request after reviewing or modifying the conflicting resource.
413 - Payload Too Large
The 413 Payload Too Large error occurs when the client sends a request that is too large for the server to process. This typically happens when the size of the request body (such as file uploads or large JSON objects) exceeds the server's configured limit.
Common Causes:
- Large file uploads: Attempting to upload files that exceed the server’s maximum allowable size.
- Oversized JSON data: Sending large payloads in API requests, such as deeply nested or voluminous JSON data, that the server cannot handle.
- Improper content-length headers: Failing to specify the content length correctly in the header can lead the server to reject the request due to size miscalculations.
Handling Strategies:
- Implement size limits on the client side: Before sending a request, ensure the data payload falls within the allowable size by configuring client-side validation.
- Use file compression: Compress large files before uploading to reduce the size and minimize the chance of triggering the error.
- Increase server limits (if appropriate): On the server side, administrators can adjust the maximum request size limits in the configuration to accommodate larger payloads.
- Split data into smaller chunks: For APIs handling large datasets, consider breaking up the data into smaller pieces and sending them over multiple requests.
415 - Unsupported Media Type
The 415 Unsupported Media Type error occurs when the server refuses to process the request because the payload's format or type is not supported. This typically happens when the content type specified in the Content-Type header is not recognized or accepted by the server.
Common Causes:
- Incorrect content type header: Sending a request with a Content-Type header that the server does not support, such as sending application/xml when the server expects application/json.
- Unsupported file format: Trying to upload a file type that the server does not accept (e.g., attempting to upload a .docx file when only .pdf files are allowed).
- Mismatched data encoding: Using a character encoding format that the server is not configured to handle, which can trigger this error.
Handling Strategies:
- Check API documentation for supported content types: Always verify the content types that the server supports and ensure the Content-Type header matches.
- Convert data to an accepted format: Before sending the request, convert the payload to a format that the server can handle, such as converting XML to JSON if needed.
- Properly configure clients to set the right content type: Ensure your client application sets the appropriate Content-Type header in requests, especially when working with file uploads or API requests that involve different data formats.
422 - Unprocessable Entity (WebDAV)
The 422 Unprocessable Entity error occurs when the server understands the content type of the request and its syntax is correct, but it’s unable to process the contained instructions. This is commonly encountered when dealing with validation errors in APIs.
Common Causes:
- Invalid data input: The client sends data that fails to meet validation rules, such as invalid format, out-of-range values, or mismatched data types.
- Missing or incorrect fields: Required fields are not provided, or fields contain incorrect data, causing the server to reject the request.
- Inconsistent resource state: The server cannot process the request because it conflicts with the current state of the resource.
Handling Strategies:
- Validate input before submission: Ensure all fields are properly filled and meet the validation requirements set by the server.
- Return detailed error messages: The server should provide specific information about which field(s) caused the failure, helping the client correct the issue.
- Use automated testing tools: Use tools to test the API payloads thoroughly, making sure they pass validation before sending them to the server.
429 - Too Many Requests
The 429 Too Many Requests error indicates that the client has exceeded the rate limit imposed by the server, usually in APIs. This limit is often put in place to prevent abuse or overuse of server resources.
Common Causes:
- Rate limit exceeded: The client is sending too many requests in a short time, often exceeding the allowed quota in rate-limited environments like public APIs.
- Bot or automated scripts: Automated tools or bots making rapid requests without proper rate-limiting logic can trigger a 429 error.
- API misconfiguration: Incorrect client configurations that ignore server rate limits or retry failed requests too quickly can result in this error.
Handling Strategies:
- Implement backoff strategies: Use strategies like exponential backoff to delay repeated requests when the rate limit is approached.
- Monitor request usage: Keep track of API calls to ensure your client stays within the allowed request limits.
- Check the Retry-After header: Many APIs provide this header, informing the client when to retry the request. Respect this information to avoid further 429 errors.
499 - Client Closed Request (Nginx-specific)
The 499 Client Closed Request is a client-side error specific to Nginx servers, indicating that the client terminated the connection before the server could complete its response. It’s a non-standard code but is often used in logging and monitoring systems to track client-side connection drops.
Common Causes:
- Premature connection closing: The client disconnects before receiving the full server response, often due to impatience or poor network conditions.
- Timeout on the client-side: A client timeout setting might be too short, causing it to close the connection while the server is still processing the request.
- Network instability: Intermittent network issues, such as packet loss or high latency, can cause the client to drop the connection.
Handling Strategies:
- Increase client-side timeout values: Adjust timeout settings on the client to allow sufficient time for the server to respond.
- Monitor network performance: Ensure network stability and bandwidth to minimize dropped connections.
- Gracefully handle client-side cancellations: Design the client to properly close requests and provide feedback to the user when a request is taking too long, rather than abruptly terminating the connection.
5xx Error Codes
500 - Internal Server Error
The 500 Internal Server Error is a generic error that occurs when the server encounters an unexpected condition that prevents it from fulfilling the request. This error can have various causes, making it one of the more challenging issues to diagnose and fix.
Common Causes:
- Server misconfigurations: Improper setup or server settings (e.g., file permission issues, memory overload).
- Application-level bugs: Unhandled exceptions, code errors, or runtime failures in the application logic.
- Database connection failures: Problems connecting to the database or timeouts can lead to a 500 error.
- Third-party service failures: If an external service or API is integrated into the system and it fails, the server might return a 500 error.
Handling Strategies:
- Review server logs: Inspect logs to identify the root cause of the error, as 500 errors often require specific information from logs.
- Monitor server health: Keep track of memory, CPU, and database usage to avoid server overloads and performance bottlenecks.
- Graceful error handling: Ensure your application handles exceptions properly and returns user-friendly error messages to avoid exposing sensitive information.
501 - Not Implemented
The 501 Not Implemented error is returned when the server does not support the functionality required to fulfill the request. It indicates that the server either lacks the capability to process the request method or that the functionality is intentionally unimplemented.
Common Causes:
- Unsupported HTTP methods: The server does not support the HTTP method being used in the request (e.g., PUT or PATCH).
- Incomplete or experimental API functionality: If a specific feature or API endpoint is still under development or intentionally disabled, a 501 error might be returned.
Handling Strategies:
- Update server capabilities: Ensure that the server supports the requested method and that all required functionality is implemented.
- Check API documentation: Verify that the API or server supports the methods being used and that they are properly configured.
- Communicate incomplete features: If certain features are not yet available, provide clear communication to the user or client indicating the limitations.
502 - Bad Gateway
A 502 Bad Gateway error occurs when a server acting as a gateway or proxy receives an invalid response from an upstream server. It usually happens in setups where multiple servers communicate with each other.
Common Causes:
- Upstream server is down: The server that the proxy or gateway is trying to reach is offline or unreachable.
- DNS issues: Problems resolving the upstream server’s IP address due to DNS misconfiguration.
- Gateway timeout: The upstream server takes too long to respond, leading the gateway server to terminate the connection.
- Server overload: The upstream server is under heavy load and unable to handle the request.
Handling Strategies:
- Check upstream server health: Ensure the upstream server is operational and can handle incoming requests.
- Verify network connectivity: Check the network path between the gateway and upstream server to ensure stable communication.
- Review DNS settings: Verify that DNS settings for the upstream server are properly configured and resolving correctly.
503 - Service Unavailable
The 503 Service Unavailable error is returned when the server is temporarily unable to handle the request, typically due to maintenance or overload. This error suggests that the server is down for a specific, often temporary, reason.
Common Causes:
- Server overload: High traffic or resource-intensive processes that exhaust server capacity can trigger a 503 error.
- Scheduled maintenance: The server is temporarily unavailable due to updates, deployments, or other planned downtime.
- Service dependency failure: A required service (like a database or external API) may be unavailable, causing the application to fail.
Handling Strategies:
- Implement proper load balancing: Use load balancers to distribute traffic and prevent server overload during peak times.
- Graceful maintenance mode: Provide a maintenance page or message during planned downtime to inform users of the server’s status.
- Monitor server performance: Use monitoring tools to track server load and identify potential overloads before they lead to a 503 error.
504 - Gateway Timeout
The 504 Gateway Timeout error occurs when a server acting as a gateway or proxy does not receive a timely response from the upstream server. Similar to 502 errors, 504 errors indicate issues with the upstream server, but specifically due to slow response times.
Common Causes:
- Slow upstream server: The upstream server is slow to respond or is taking longer than the gateway’s timeout limit.
- Network latency: High latency between the proxy server and the upstream server can lead to timeout errors.
- Server overload: The upstream server is under heavy load and unable to respond within the allocated time.
Handling Strategies:
- Optimize upstream server performance: Ensure that the upstream server is optimized and not overloaded with requests.
- Adjust timeout settings: Increase the gateway’s timeout limit to accommodate longer response times, especially for slow services.
- Reduce latency: Ensure that network connections between servers are optimized to minimize latency and reduce timeout errors.
HTTP Redirect Codes
HTTP redirect codes (3xx) are used to indicate that the requested resource has been temporarily or permanently moved to another location. When a client, such as a browser or API client, encounters a 3xx status code, it knows that it must take additional steps (usually following the new URL) to complete the request. Redirects are essential for tasks like URL restructuring, changing domain names, and load balancing.
301 - Moved Permanently
A 301 Moved Permanently status code means that the resource requested has been permanently moved to a new URL. After receiving a 301 response, clients (browsers or search engines) will automatically update their records to use the new URL for future requests. This type of redirect is commonly used when websites restructure or change domains.
When and Why to Use:
- SEO best practices: 301 redirects are crucial for maintaining search engine rankings when changing URLs, as they pass link equity (SEO value) from the old URL to the new one.
- Permanent URL changes: Use a 301 redirect when a resource has been permanently moved, such as moving a website to a new domain or restructuring the URL hierarchy.
- User experience: Ensure users who bookmarked or linked to the old URL are seamlessly redirected to the new location.
302 - Found (Temporary Redirect)
A 302 Found status code indicates that the resource has been temporarily moved to a different URL, but the original URL should still be used for future requests. This tells the client that the change is only temporary, and no need to update bookmarks or search engine records.
When and Why to Use:
- Temporary content moves: Use a 302 redirect when content is temporarily moved to another location, such as during maintenance or A/B testing.
- Preserve original URL: Since the change is temporary, a 302 ensures that the original URL remains valid for the long term, which is important if the resource will eventually return to the original location.
- Testing or redirection for specific user groups: For example, you might temporarily redirect users from one region to a specific version of a page, while keeping the original for others.
Error Codes in REST APIs
In API development, handling error codes effectively is crucial for delivering a robust and developer-friendly experience. HTTP status codes serve as a key communication tool between the server and the client, allowing developers to understand the outcome of an API request. While success codes (2xx) indicate a successful transaction, error codes provide insights into failures and help identify what went wrong.
Importance of Error Codes in API Responses
When developing or consuming APIs, error codes help streamline troubleshooting by signaling the exact nature of the problem. A well-defined error response can guide developers toward the specific issue, whether it's due to client-side mistakes, server failures, or connectivity problems. Clear and meaningful error codes enhance the overall API documentation and developer experience.
Handling and Interpreting These Codes in API Development
- Client-Side Errors (4xx): These errors indicate that the issue lies on the client side, such as malformed requests or unauthorized access attempts. For example, a 400 Bad Request error could result from sending improperly formatted data, while a 401 Unauthorized error suggests missing or invalid authentication credentials.
- Server-Side Errors (5xx): Server-side issues typically involve problems that prevent the server from fulfilling valid requests. These might include server overload (503 Service Unavailable) or system errors (500 Internal Server Error).
- Best Practices for Returning Meaningful Error Messages:
- Descriptive error responses: Include a detailed error message with the HTTP status code that explains the reason for the failure (e.g., missing fields or unsupported media types).
- Error codes in JSON format: For APIs returning JSON, structure error messages in a predictable format (e.g., with fields like "error_code" and "message").
- Link to documentation: Provide links in the error response that direct developers to the relevant documentation or troubleshooting guides.
- Consistency: Ensure that error responses across the API follow a consistent structure and format, making it easier for developers to handle errors uniformly.
FAQs
Here are some frequently asked questions related to HTTP error codes, especially in the context of web and API development:
1. What are HTTP Error Codes?
HTTP error codes are standard response codes returned by servers to indicate the result of a client’s request. They help both developers and users understand whether a request was successfully processed or if there was an issue, such as an invalid request or server failure. These codes are divided into categories like 2xx (successful requests), 3xx (redirections), 4xx (client-side errors), and 5xx (server-side errors).
2. What is HTTP code 302 Found?
The 302 Found status code is a redirection message. It indicates that the requested resource is temporarily located at a different URL, and the client should use the new location provided in the response to fetch the resource. This redirection is temporary, meaning the original URL is still expected to be valid in the future.
3. What do 200, 403, and 503 HTTP error codes mean?
- 200 OK: The request has succeeded. This is the standard response for successful HTTP requests.
- 403 Forbidden: The server understands the request but refuses to authorize it, often due to insufficient permissions or authentication failures.
- 503 Service Unavailable: The server is temporarily unable to handle the request, typically due to overload or maintenance, and should return to normal service after some time.
4. How can clearing the browser's cache resolve HTTP errors?
Clearing the browser's cache can resolve issues related to an outdated or corrupted cache, which might lead to errors like 400 Bad Request or 'HTTP Error 401.' An expired or corrupted cache can cause unexpected behavior and specific errors. To troubleshoot these errors effectively, it's important to clear the outdated browser cache and cookies. This process varies across different browsers, but generally, you can find the option to clear the cache in the browser's settings or preferences menu.
4. Which HTTP error codes would indicate a redirect?
Redirect codes fall under the 3xx category. Common redirect codes include:
- 301 Moved Permanently: The requested resource has been permanently moved to a new URL.
- 302 Found: The resource is temporarily located at a different URL.
- 307 Temporary Redirect: The resource is temporarily located at a different URL, and the method used for the request should not change.
5. What is HTTP error code 405?
The 405 Method Not Allowed error indicates that the method used in the request (e.g., GET, POST) is not supported by the server for the requested resource. This often happens when the server expects a specific method (like POST) but receives a different one (like GET).
6. How to fix the 302 status code in API request?
A 302 status code may appear in API responses when there is a temporary redirect. To resolve it:
- Check API documentation to confirm the correct endpoint is being used.
- Ensure proper redirection handling: Some APIs might expect clients to follow redirects automatically.
- Avoid unnecessary redirects: Review the server logic to ensure that redirects are used only when needed. If the redirect is unintentional, consider fixing the logic to return a more appropriate status code, like 200 OK.
Summary
Mastering HTTP error codes is crucial for web and API development, as these codes offer insights into how requests are processed between clients and servers. By understanding key 4xx client-side errors, 5xx server-side errors, and essential redirect codes, developers can quickly diagnose issues, improve user experience, and optimize application performance. Whether you’re handling invalid requests, API timeouts, or server overloads, this knowledge equips you to resolve issues effectively.
Ready to streamline your API development? Sign up at API Freaks today, get your free API key, and access a powerful collection of APIs for seamless integration and innovation.